How Builders Can Make improvements to Their Debugging Techniques By Gustavo Woltmann
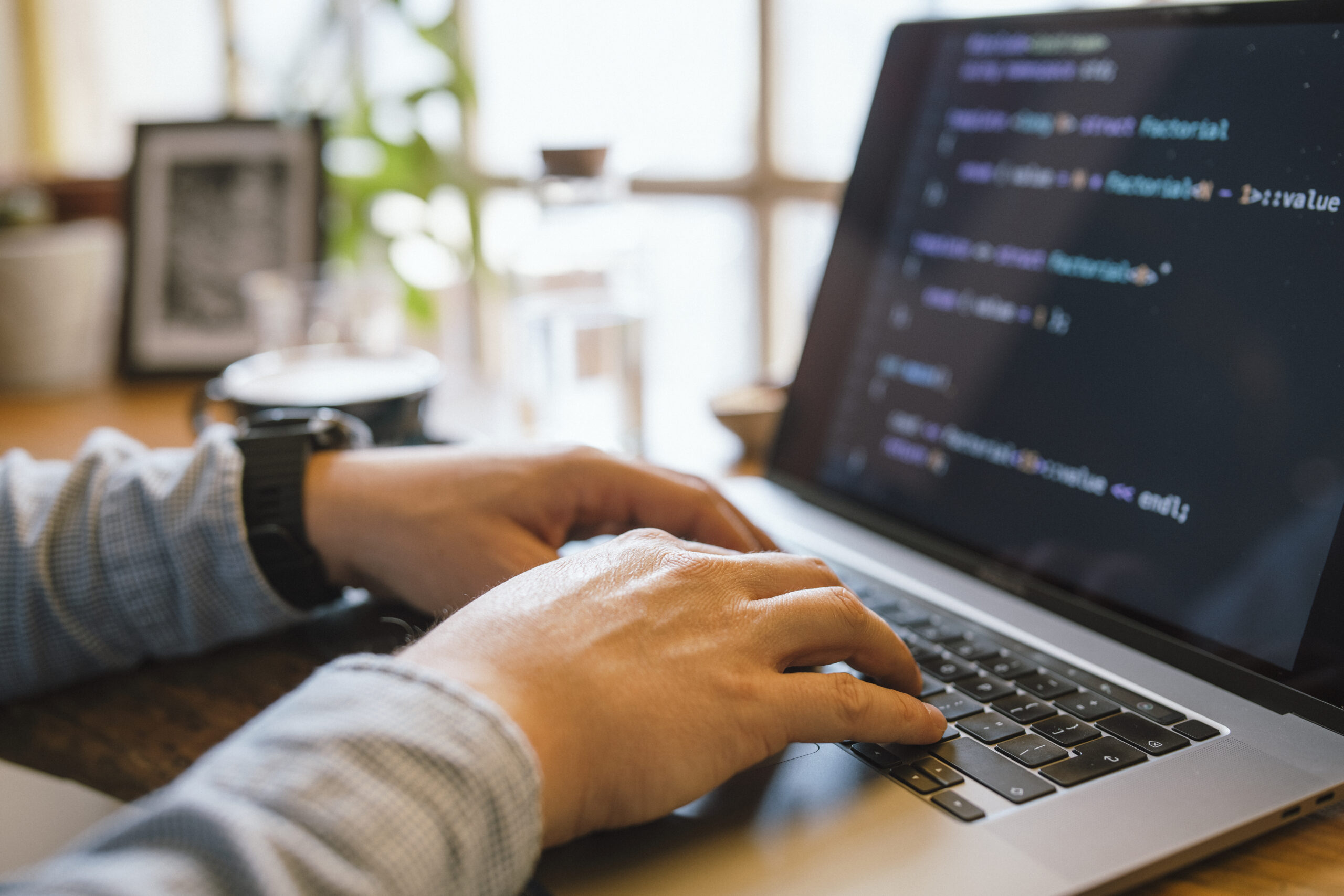
Debugging is Among the most essential — nevertheless generally overlooked — abilities within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Finding out to Assume methodically to unravel challenges competently. Whether you're a beginner or a seasoned developer, sharpening your debugging skills can save hours of frustration and considerably help your efficiency. Here i will discuss quite a few strategies that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. Though producing code is one particular Portion of development, recognizing tips on how to communicate with it successfully during execution is Similarly crucial. Contemporary enhancement environments appear equipped with impressive debugging abilities — but lots of developers only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, and even modify code to the fly. When employed properly, they Allow you to notice just how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, check out authentic-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command systems like Git to comprehend code historical past, come across the precise instant bugs were launched, and isolate problematic improvements.
Finally, mastering your tools indicates going further than default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not shed at the hours of darkness. The greater you are aware of your tools, the greater time you could spend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the situation
Among the most essential — and sometimes disregarded — measures in efficient debugging is reproducing the condition. Right before leaping to the code or creating guesses, developers have to have to make a steady surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting as much context as is possible. Request concerns like: What steps resulted in The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it becomes to isolate the precise ailments below which the bug takes place.
After you’ve gathered adequate information, try and recreate the problem in your neighborhood environment. This might mean inputting precisely the same data, simulating related user interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated exams that replicate the sting cases or condition transitions associated. These tests not merely assistance expose the trouble and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on sure operating techniques, browsers, or underneath individual configurations. Utilizing resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You should use your debugging resources a lot more properly, take a look at probable fixes safely and securely, and converse additional Plainly with the staff or end users. It turns an summary grievance right into a concrete problem — and that’s in which developers thrive.
Read through and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders need to understand to treat error messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and sometimes even why it took place — if you understand how to interpret them.
Begin by examining the concept very carefully and in whole. Several builders, particularly when under time force, glance at the first line and straight away commence creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a particular file and line range? What module or perform brought on it? These concerns can tutorial your investigation and stage you toward the liable code.
It’s also beneficial to be familiar with the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Mastering to acknowledge these can greatly quicken your debugging course of action.
Some errors are obscure or generic, As well as in Those people instances, it’s critical to look at the context by which the error transpired. Look at associated log entries, input values, and up to date variations in the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger concerns and supply hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging concentrations involve DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common occasions (like successful get started-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your method. Focus on important situations, condition modifications, enter/output values, and demanding decision factors in your code.
Structure your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log more info rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging technique, you can reduce the time it takes to identify issues, achieve further visibility into your applications, and Enhance the Total maintainability and trustworthiness of your code.
Consider Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To properly establish and fix bugs, developers will have to method the method just like a detective solving a mystery. This way of thinking allows break down intricate challenges into workable components and stick to clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the signs or symptoms of the condition: mistake messages, incorrect output, or performance concerns. Similar to a detective surveys a criminal offense scene, accumulate just as much relevant information as you are able to without having jumping to conclusions. Use logs, exam cases, and person experiences to piece alongside one another a transparent photograph of what’s occurring.
Following, sort hypotheses. Question by yourself: What may be triggering this conduct? Have any modifications lately been produced to the codebase? Has this issue happened in advance of beneath equivalent situations? The goal should be to slender down opportunities and recognize potential culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge within a controlled ecosystem. When you suspect a particular function or part, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Shell out close awareness to tiny details. Bugs generally conceal during the minimum envisioned spots—like a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may well hide the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and assistance Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed difficulties in complex techniques.
Produce Checks
Writing exams is one of the best tips on how to improve your debugging expertise and Total progress performance. Tests not only enable capture bugs early but will also function a security net that gives you assurance when making modifications in your codebase. A properly-examined software is simpler to debug as it lets you pinpoint particularly where by and when a dilemma takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a test fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These assistance be sure that a variety of elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complex devices with several components or expert services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the check fails continually, you are able to center on fixing the bug and observe your exam go when the issue is settled. This tactic ensures that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable system—assisting you catch far more bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, making an attempt Resolution just after Alternative. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are far too near to the code for way too very long, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. On this point out, your Mind results in being fewer successful at challenge-fixing. A short walk, a espresso crack, or maybe switching to a distinct process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly all through extended debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Electricity as well as a clearer state of mind. You may perhaps out of the blue recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
If you’re caught, a good general guideline would be to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver about, stretch, or do a little something unrelated to code. It might feel counterintuitive, Specially under restricted deadlines, but it in fact leads to speedier and more effective debugging In the long term.
In brief, getting breaks is not an indication of weak spot—it’s a wise tactic. It provides your Mind House to breathe, enhances your standpoint, and assists you steer clear of the tunnel eyesight that often blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Understand From Each Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing worthwhile when you take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a number of critical issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like device tests, code reviews, or logging? The responses often expose blind places in the workflow or being familiar with and help you build stronger coding habits moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but individuals that continually learn from their problems.
Eventually, Each and every bug you take care of adds a fresh layer towards your skill established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging competencies requires time, exercise, and tolerance — however the payoff is big. It will make you a more productive, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s a chance to become superior at what you do.